Topics
Introduction
I have used Swift for windows 1.9 for debugging purpose. But you can use any Swift programming language compiler as per your availability.
func insert_string(_ str1: String, _ str2: String) -> String { var current_index = str1.startIndex let char1: Character = str1[current_index] var result = str1 while char1 == str1[current_index] { current_index = str1.index(after: current_index) } result.insert(contentsOf: str2.characters, at: current_index) return result } print(insert_string("<>", "Swift")) print(insert_string("<>>", "Swift")) print(insert_string("[]", "Swift"))
Result
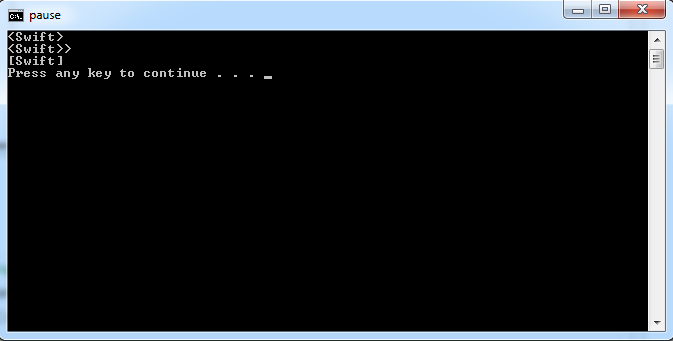