Introduction
C program to input roll number, student name and marks of three subjects (Physics, Chemistry and Information Technology) and calculate total marks, percentage and division of student. I have used DEV-C++ compiler for debugging purpose. But you can use any C programming language compiler as per your availability.
#include <stdio.h> #include <string.h> void main() { int rollno, phy, che, it, total; float percentage; char name[20], div[10]; printf("Input the Roll Number of the student :"); scanf("%d", &rollno ); printf("Input the Name of the Student :"); scanf("%s", name); printf("Input the marks of Physics, Chemistry and Information Technology: "); scanf("%d%d%d", &phy, &che, &it); total = phy + che + it; percentage = total/3.0; if (percentage >= 60) strcpy(div, "First"); else if (percentage < 60 && percentage >= 48) strcpy(div,"Second"); else if (percentage <48 && percentage >= 36) strcpy(div, "Pass"); else strcpy(div, "Fail"); printf("\nRoll No : %d\nName of Student : %s\n", rollno, name); printf("Marks in Physics : %d\nMarks in Chemistry : %d\nMarks in Information Technology : %d\n", phy, che, it); printf("Total Marks = %d\nPercentage = %5.2f\nDivision = %s\n", total, percentage, div); }
Result
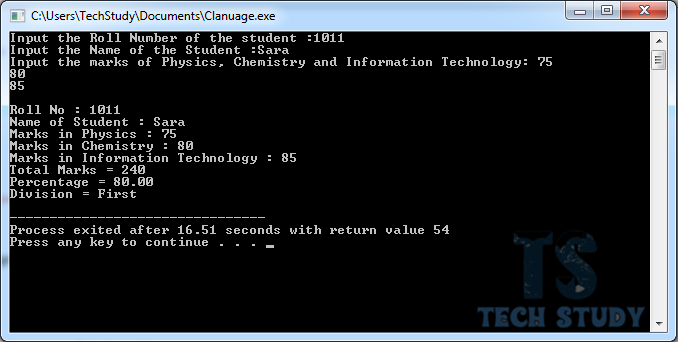