Introduction
Write C# Program to Check whether an alphabet is a vowel or not. I have used Visual Studio 2012 for debugging purpose. But you can use any version of visul studio as per your availability..
using System; public class exercise17 { static void Main(string[] args) { char ch; Console.Write("Input an Alphabet (A-Z or a-z) : "); ch = Convert.ToChar(Console.ReadLine().ToLower()); int i = ch; if (i >= 48 && i <= 57) { Console.Write("You entered a number, Please enter an alpahbet."); } else { switch (ch) { case 'a': Console.WriteLine("The Alphabet is vowel"); break; case 'i': Console.WriteLine("The Alphabet is vowel"); break; case 'o': Console.WriteLine("The Alphabet is vowel"); break; case 'u': Console.WriteLine("The Alphabet is vowel"); break; case 'e': Console.WriteLine("The Alphabet is vowel"); break; default: Console.WriteLine("The Alphabet is not a vowel"); break; } } Console.ReadLine(); } }
Result
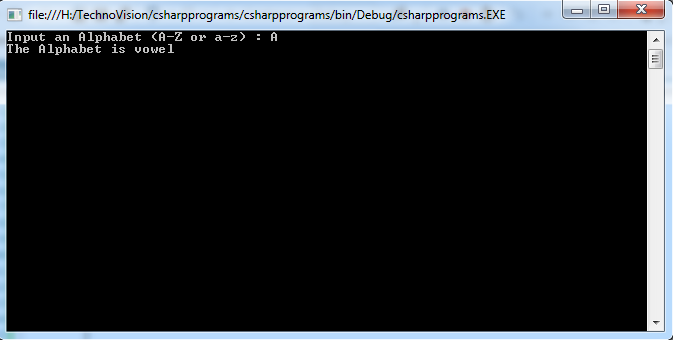
Console.Write(“You entered a number, Please enter an alpahbet.”);
typo. (alphabet)
when you run the code and input an alphabet which is not a vowel there is no output to show that it is not a vowel
class Town
{
static void Main()
{
char ch;
Console.WriteLine(“Input alphabet A-Z or a-z”);
ch = Convert.ToChar(Console.ReadLine().ToLower());
int i = ch;
if(i>=48 && i<=57)
{
Console.WriteLine("please enter an alaphabet");
}
else
{
switch(ch)
{
case 'a':
Console.WriteLine("Is a vowel");
break;
case 'e':
Console.WriteLine("Is a vowel");
break;
case 'i':
Console.WriteLine("Is a vowel");
break;
case 'o':
Console.WriteLine("Is a vowel");
break;
case 'u':
Console.WriteLine("Is a vowel");
break;
case 'b' :
Console.WriteLine("Is not a vowel");
break;
case 'c':
Console.WriteLine("Is not a vowel");
break;
case 'd':
Console.WriteLine("Is not a vowel");
break;
case 'f':
Console.WriteLine("Is not a vowel");
break;
case 'g':
Console.WriteLine("Is not a vowel");
break;
case 'h':
Console.WriteLine("Is not a vowel");
break;
case 'j':
Console.WriteLine("Is not a vowel");
break;
case 'k':
Console.WriteLine("Is not a vowel");
break;
case 'l':
Console.WriteLine("Is not a vowel");
break;
case 'm':
Console.WriteLine("Is not a vowel");
break;
case 'n':
Console.WriteLine("Is not a vowel");
break;
case 'p':
Console.WriteLine("Is not a vowel");
break;
case 'q':
Console.WriteLine("Is not a vowel");
break;
case 'r':
Console.WriteLine("Is not a vowel");
break;
case 's':
Console.WriteLine("Is not a vowel");
break;
case 't':
Console.WriteLine("Is not a vowel");
break;
case 'v':
Console.WriteLine("Is not a vowel");
break;
case 'w':
Console.WriteLine("Is not a vowel");
break;
case 'x':
Console.WriteLine("Is not a vowel");
break;
case 'y':
Console.WriteLine("Is not a vowel");
break;
case 'z':
Console.WriteLine("Is not a vowel");
break;
}
}
Console.ReadKey();
}
}