Topics
Introduction
Write C program to find HCF of two numbers.
What is HCF?
Highest Common Factor (H.C.F.) of two natural numbers is the largest common factor (or divisor) of the given natural numbers. The Highest Common Factor is also known as the greatest common factor (gcf).
For example, the hcf of 54 and 24 is 6
#include <stdio.h> int main() { int i, num1, num2, min, HCF=1; //Read two numbers from user printf("Enter any two numbers: "); scanf("%d%d", &num1, &num2); // Find min number between two numbers min = (num1<num2) ? num1 : num2; for(i=1; i<=min; i++) { if(num1%i==0 && num2%i==0) { HCF = i; } } printf("HCF of %d and %d = %d\n", num1, num2, HCF); return 0; }
Result
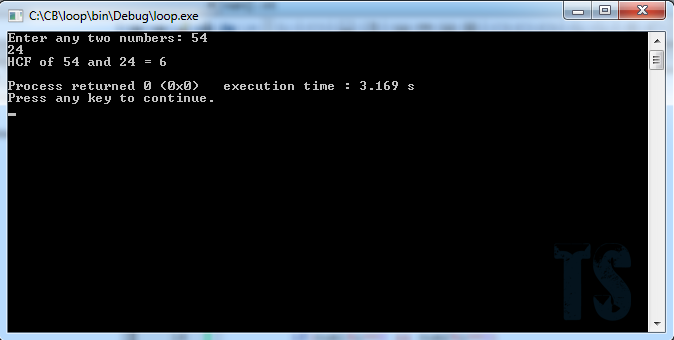