Introduction
I have used Visual Studio 2012 for debugging purpose. But you can use any version of visul studio as per your availability..
using System; using System.Collections.Generic; using System.Linq; using System.Text; public class csharpExercise { static void Main(string[] args) { int num, last, first, temp, count = 0; double swap; // Reading number Console.Write("Enter any number: "); num = Convert.ToInt32(Console.ReadLine()); temp = num; last = temp % 10; count = (int)Math.Log10(temp); while (temp >= 10) { temp /= 10; } first = temp; swap = (last * Math.Pow(10, count) + first) + (num - (first * Math.Pow(10, count) + last)); Console.WriteLine("Last Digit:" + last); Console.WriteLine("First Digit:" + first); Console.WriteLine(num + " is swapped to " + swap); Console.ReadLine(); } }
Result
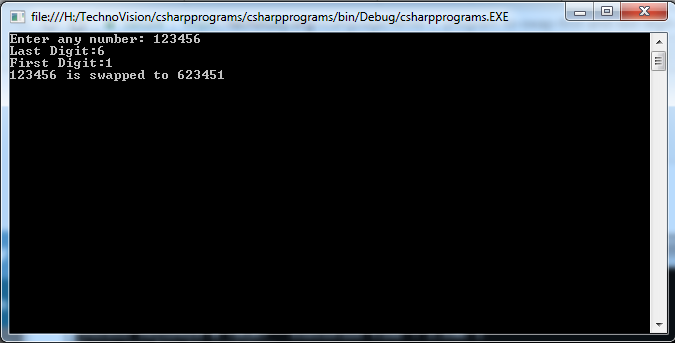