Introduction
Write C# Program to find the Largest among Three Variables using Nested if. I have used Visual Studio 2012 for debugging purpose. But you can use any version of visul studio as per your availability..
using System; namespace csharpprograms { class Program { static void Main(string[] args) { int num1, num2, num3; Console.WriteLine("Enter three numbers: \n"); num1 = Convert.ToInt32(Console.ReadLine()); num2 = Convert.ToInt32(Console.ReadLine()); num3 = Convert.ToInt32(Console.ReadLine()); if (num1 >= num2) { if (num1 >= num3) Console.WriteLine(num1+" is the largest number"); else Console.WriteLine(num3 + " is the largest number"); } else if (num2 >= num3) Console.WriteLine(num2 + " is the largest number"); else Console.WriteLine(num3 + " is the largest number"); Console.ReadLine(); } } }
Result
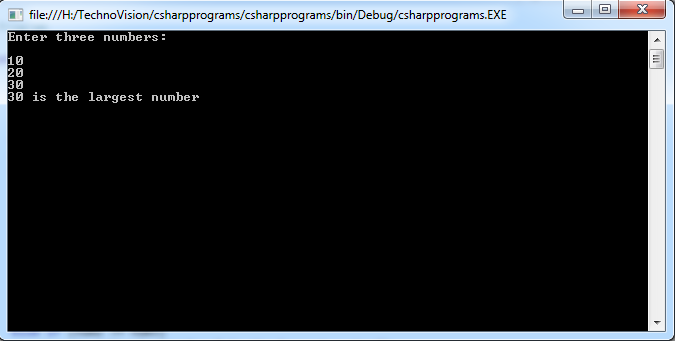