Introduction
I have used CodeBlocks compiler for debugging purpose. But you can use any C++ programming language compiler as per your availability.
#include <iostream> using namespace std; int main() { int a[10][10], b[10][10], result[10][10], r1, c1, r2, c2, i, j, k; cout<<"Enter rows and column for first matrix: "; cin>>r1; cin>>c1; cout<<"Enter rows and column for second matrix: "; cin>>r2; cin>>c2; // Column of first matrix should be equal to column of second matrix and while (c1 != r2) { cout<<"Error! column of first matrix not equal to row of second.\n\n"; cout<<"Enter rows and column for first matrix: "; cin>>r1; cin>>c1; cout<<"Enter rows and column for second matrix: "; cin>>r2; cin>>c2; } // Storing elements of first matrix. cout<<"\nEnter elements of matrix 1:\n"; for(i=0; i<r1; ++i) for(j=0; j<c1; ++j) { cout<<"Enter element a"<<i+1<<j+1<<": "; cin>>a[i][j]; } // Storing elements of second matrix. cout<<"\nEnter elements of matrix 2:\n"; for(i=0; i<r2; ++i) for(j=0; j<c2; ++j) { cout<<"Enter element b"<<i+1<<j+1<<": "; cin>>b[i][j]; } // Initializing all elements of result matrix to 0 for(i=0; i<r1; ++i) for(j=0; j<c2; ++j) { result[i][j] = 0; } // Multiplying matrices a and b and // storing result in result matrix for(i=0; i<r1; ++i) for(j=0; j<c2; ++j) for(k=0; k<c1; ++k) { result[i][j]+=a[i][k]*b[k][j]; } // Displaying the result cout<<"\nOutput Matrix:\n"; for(i=0; i<r1; ++i) for(j=0; j<c2; ++j) { cout<<" "<<result[i][j]; if(j == c2-1) cout<<"\n\n"; } return 0; }
Result
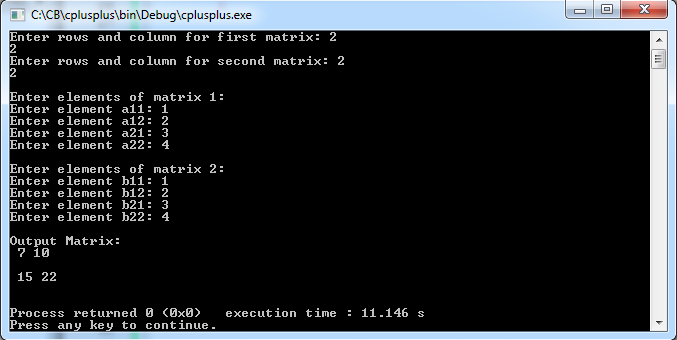